Implementing Facebook login / single sign-on (part 1)
I am implementing Facebook single sign on for my applications. In this first part of my Facebook authentication tutorial, I discuss the basics of the Facebook authentication process (see also: part 2, part 3).
Getting started: some terminology
If you are like me and haven't implemented Facebook integration before, the terminology and various API's can be confusing. And the Facebook developer documentation is a combination of too-much-information and not-enough-explanation.First, the Facebook documentation talks about different kinds of applications, which are:
- Facebook canvas applications: Applications-within-Facebook, or what users think of as "Facebook apps".
- FBML / FBJS apps (built using FB-specific markup)
- Iframe canvas apps (built using Javascript and the FB JS API)
- Facebook desktop applications (rare; basically anything that cannot run within a browser, like a desktop client for Facebook)
- Facebook web applications (websites with Facebook-integration such as single sign-on and custom Facebook elements within them such as CNN and Digg)
- Facebook Connect - no such thing exists anymore (e.g. http://mashable.com/2010/04/21/facebook-kills-facebook-connect/). The new API is called the Graph API, and talking about Facebook Connect is inaccurate..
- APIs (always used, either on the server side or on the client's web browser via Javascript)
- Graph API: The new version of Facebook's API. The Graph API is much more than just authentication: it is the mechanism which powers Facebook applications and allows you to read and write date to Facebook.
- Old REST API: The early version of Facebook's Graph API; Facebook does not recommend you use it because they are in the process of deprecating it.
- FQL (Facebook Query Language): A SQL-like language for querying the Graph API. Supposedly makes using the API easier for apps.
- Markup
- FBML (Facebook Markup Language): A HTML-like language for building Facebook applications. FBML is rendered on the Facebook server. Facebook does not recommend that you use it; instead suggesting that you use the Graph API via their Javascript SDK with XFBML.
- XFBML: FBML-within-(X)HTML pages rendered using Facebook's Javascript SDK.
- SDKs
- Language-specific libraries for querying the API. Other than the Javascript SDK, it's all server-side and based on HTTP requests.
- Note that the Javascript SDK allows you to render XFBML embedded on a (X)HTML page! This was confusing because I had done something with FBML before, and didn't see where exactly the transformation from fb:login-button to HTML was occurring...
- Other stuff from Facebook:
- Open Graph Protocol: A convention of meta and other tags which allows you to add metadata to Like button clicks, integrating them with Facebook (e.g. a like on a profile).
- Social plugins: Ready-made Facebook widgets you can embed using an iframe.
How does Facebook authentication work?
Conceptually, it works like this (picture of user represents page shown to user):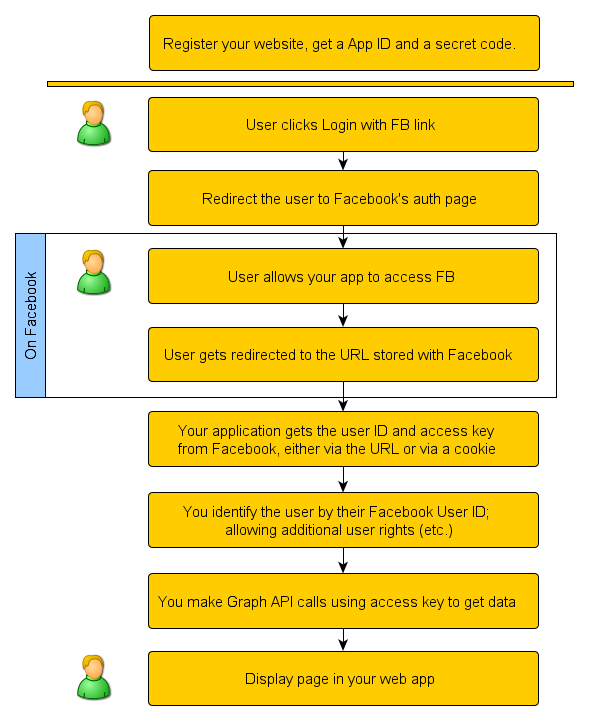
- Javascript single sign-on: The Javascript single sign-on is simplest, because it provides a function (FB.login) which handles everything from the details of OAuth 2.0 requests to rendering the actual login button. When using the Javascript API, the user id and access token are stored in a cookie (e.g. fbs_app_id) which you can access both server-side and via Javascript in the client.
- web application authentication (using server side SDK's): When you do web application authentication, you need redirect the user to Facebook in your app. When the user allows access for your app, Facebook redirects them back and sends the access token and user ID as part of the GET request to your authentication-complete redirection page. Then you can access the user's information using the returned token.
Next part
In part 2 of my Facebook login tutorial I address database design considerations and look at how Facebook login can be integrated with your existing user management. In part 3 of my Facebook login tutorial I go into implementation specifics using the Javascript SDK. References- http://developers.facebook.com/docs/
- http://developers.facebook.com/docs/guides/performance (good illustration on the difference between FBML and IFrame applications)
- http://stackoverflow.com/questions/1175047/facebook-app-vs-facebook-connect-site
Comments
Alex: Thanks for creating this. I am also confused about the ever-changing technologies FB is using. I wish they made it simpler. Your post helped me understand a bunch of different nuances. :)
Grant: Thank you. It's often only in seeing all of the pieces side by side that we understand. I hadn't found something so clear and comprehensive as your post.
don11: thanks. if the user logged out and or the cookies cleared. the access token will became invalid. what is the best way to handle that